Date Manipulation in T-SQL: A Deep-Dive on DATEADD
- MikeBennyhoff
- Feb 25, 2024
- 6 min read
Updated: Mar 7, 2024
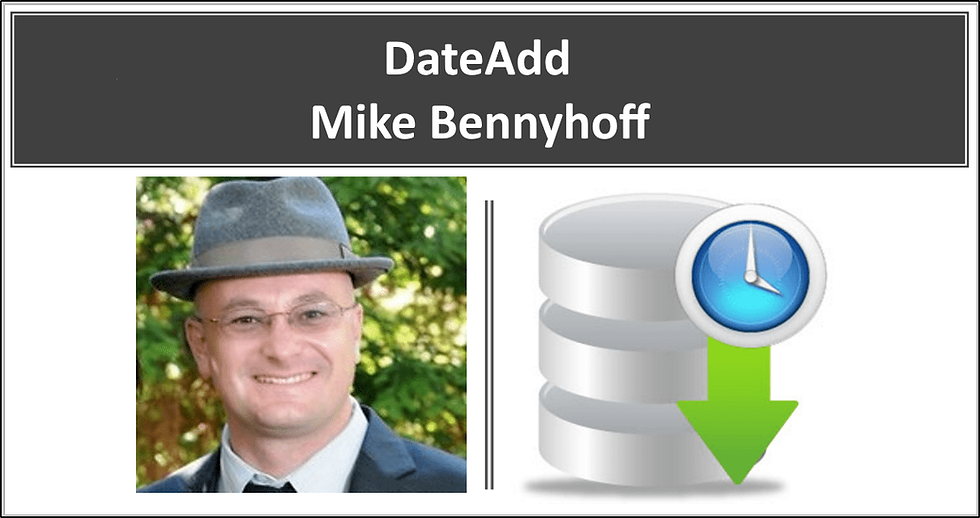
For SQL developers and database administrators, mastery over Date Manipulation in T-SQL: A Deep-Dive on DATEADD is as critical as understanding the SELECT or JOIN commands. One of the pillars of temporal operations in Transact-SQL (T-SQL) is the DATEADD function, a powerful tool for adjusting date and time values. In this comprehensive guide, we will explore the ins and outs of DATEADD in T-SQL and how it can enhance your data querying and analysis capabilities.
The Significance of DATEADD in T-SQL
The DATEADD function is a T-SQL feature designed to add or subtract a specified number or time interval from a given date. This can be crucial when you need to perform complex calculations, such as determining deadlines, aging assets, working with fiscal year data, and more. Not only does it give you the flexibility to manipulate dates effectively, but it is also a valuable ally in crafting queries that reflect dynamic time-based conditions.
The SQL Server DATEADD function is used to add or subtract a specified time interval (such as days, months, years, etc.) to a given date. Here are some examples demonstrating its usage:
Adding Days to a Date From An Input Date Value
DECLARE @StartDate DATETIME = '2024-02-15';
SELECT DATEADD(DAY, 7, @StartDate) AS NewDate;
This example function adds 7 days to the @StartDate and returns the resulting date.
Subtracting Months from a Date:
DECLARE @StartDate DATETIME = '2024-06-15';
SELECT DATEADD(MONTH, -3, @StartDate) AS NewDate;
Here, 3 months are subtracted from the @StartDate.
Adding Years to a Date:
DECLARE @StartDate DATETIME = '2024-02-15';
SELECT DATEADD(YEAR, 2, @StartDate) AS NewDate;
This example adds 2 years to the @StartDate.
Adding Hours, Minutes, and Seconds:
DECLARE @StartTime DATETIME = '2024-02-15 09:30:00';
SELECT DATEADD(HOUR, 3, @StartTime) AS NewTime,
DATEADD(MINUTE, 15, @StartTime) AS NewTimePlus15Min,
DATEADD(SECOND, 45, @StartTime) AS NewTimePlus45Sec;
Here, we want to add 3 hours, 15 minutes, and 45 seconds to the @StartTime.
Adding Weeks to a Date:
DECLARE @StartDate DATETIME = '2024-02-15';
SELECT DATEADD(WEEK, 2, @StartDate) AS NewDate;
Calculating business days (excluding weekends) using DATEADD in SQL Server involves adding or subtracting days while skipping Saturdays and Sundays. Here’s an example of
how you can do this:
DECLARE @StartDate DATE = '2024-02-10';
DECLARE @NumOfBusinessDays INT = 10; -- Number of business days to add
-- Initialize a counter for business days
DECLARE @BusinessDaysCounter INT = 0;
-- Loop through each day and count business days
WHILE @BusinessDaysCounter < @NumOfBusinessDays
BEGIN
-- Add one day to the start date
SET @StartDate = DATEADD(DAY, 1, @StartDate);
-- Check if the day is not Saturday (6) or Sunday (0)
IF DATEPART(WEEKDAY, @StartDate) NOT IN (1, 7)
BEGIN
-- Increment the counter if it's a business day
SET @BusinessDaysCounter = @BusinessDaysCounter + 1;
END;
END;
SELECT @StartDate AS EndDate;
In this example:
@StartDate is the starting date.
@NumOfBusinessDays is the number of business days you want to add.
We loop through each day starting from @StartDate and increment the current date by one day using DATEADD.
Inside the loop, we check if the date data type current day is not Saturday (6) or Sunday (0) using DATEPART(WEEKDAY).
If the day is a business day (not Saturday or Sunday, following example), we increment to use the dateadd @BusinessDaysCounter.
Once the counter reaches @NumOfBusinessDays, we exit the loop, and @StartDate holds the new column end date after adding the specified number of business days.
This approach ensures that only weekdays (Monday through Friday) are counted as business days. Adjustments may be needed for holidays depending on your specific requirements.
This example adds 2 weeks to the date parameter @StartDate.
These examples illustrate how the DATEADD function can be used to manipulate data types for dates and times in SQL Server queries.
Using the SQL SERVER DATEADD function to get records from a table in specified date range
We may utilize the DATEADD SQL function to retrieve data from the database in periods or days. The following request specifies a date using parameter @Start. This can be done through the order tables. It is important to have records from startdates. (Add an hour to enddates) Date & Date= 0 00 03:00. Define time INT = 1: SELECT OrderID and Last editedWhen from [Global importer]. [Deals]. [An order] – When it’s last edited between a time and a date ADD(hors, hours, start dates).
Using the SQL SERVER DATEADD function to get date or time difference
Using DateADD the SQL function returns a time difference at a given start time. Often times a SQL server can be used for date change. For instance, we need the length of time required for the delivery or the distance to the home office. Run the following query for the difference in start time. We use the DATEDIF SQL function alongside DATINGIFF SQL. DATE – DEFECT : @StartingTime. DATE-Time. DATE-IME. 2019-04-30 01:00:00.
Return types
Return value data types are dynamic. The data type int is returned depends upon the arguments provided for Dateadd. When date values are integer dates, DATEADD returns datetime values. The date add datatype returned for date add can be supplied in a valid input form. DATAADD produces errors when string literal seconds scales are larger than three decimal positions.
Datepart Argument
The DATEPART function in T-SQL is used to extract a specific part of a date or time value. It returns an integer representing the specified date value or time part. Here are one of the following different possibilities for the DATEPART argument:
Year (yy, yyyy): Returns the year part of the input date, return month or time value.
Quarter (qq, q): Returns the quarter of the year (1 through 4) of the date or time value.
Month (mm, m): Returns the month part of the date or time value (1 through 12).
Day of Year (dy, y): Returns the day of the year (1 through 366) of the date or time value.
Day (dd, d): Returns the day of the month (1 through 31) of the date or time value.
Week (wk, ww): Returns the week number (1 through 53) of the date or time value.
Weekday (dw, w): Returns the weekday number (1 through 7) of the date or time value, where Sunday is 1 and Saturday is 7.
Hour (hh): Returns the hour part (0 through 23) of the time value.
Minute (mi, n): Returns the minute part (0 through 59) of the time value.
Second (ss, s): Returns the second part (0 through 59) of the time value.
Millisecond (ms): Returns the millisecond part (0 through 999) of the time value.
Microsecond (mcs): Returns the microsecond part (0 through 999999) of the time value.
Nanosecond (ns): Returns integer number of the nanosecond part (0 through 999999999) of the time value.
TZoffset (tz): Returns the time zone offset in minutes for the current date, or time value.
These are the possible values that you can use as the first argument in the DATEPART function to extract specific date or time components from a given table or full date value or time value in T-SQL.
Time Zone Conversions
Performing time zone conversions in T-SQL typically involves adjusting datetime values to reflect the difference between two time zones. Here’s how you can use DATEADD to perform time zone conversions, along with examples:
Example 1: Converting UTC to Local Time
DECLARE @UtcDateTime DATETIME = '2024-02-20 10:00:00';
DECLARE @TimeZoneOffset INT = DATEDIFF(MINUTE, GETUTCDATE(), GETDATE());
SELECT DATEADD(MINUTE, @TimeZoneOffset, @UtcDateTime) AS LocalDateTime;
In this example, @UtcDateTime represents a datetime value in UTC. We calculate the time zone offset between UTC and the local time zone using DATEDIFF(MINUTE, GETUTCDATE(), GETDATE()), which returns the difference in minutes. Then, we add this offset to the UTC datetime value using DATEADD to obtain the corresponding local datetime.
Example 2: Converting Local Time to UTC
DECLARE @LocalDateTime DATETIME = '2024-02-20 10:00:00';
DECLARE @TimeZoneOffset INT = DATEDIFF(MINUTE, GETUTCDATE(), GETDATE());
SELECT DATEADD(MINUTE, -@TimeZoneOffset, @LocalDateTime) AS UtcDateTime;
Here, @LocalDateTime represents a datetime value in the local time zone. We calculate the time zone offset between the date in the local time zone and UTC in minutes. Then, we subtract this offset from the local datetime value using DATEADD to obtain number date of the corresponding UTC datetime.
Example 3: Converting Between Different Time Zones
DECLARE @UtcDateTime DATETIME = '2024-02-20 10:00:00';
DECLARE @TimeZoneOffset INT = DATEDIFF(MINUTE, GETUTCDATE(), GETDATE());
DECLARE @TargetTimeZoneOffset INT = -480; -- Pacific Standard Time (PST) offset in minutes
SELECT DATEADD(MINUTE, @TargetTimeZoneOffset - @TimeZoneOffset, @UtcDateTime) AS TargetDateTime;
In this example, we convert a UTC datetime value to a different time zone (Pacific Standard Time, PST). First, we calculate the time zone offset between UTC and the local time zone.
Then, we add the difference between the target time zone offset and the local time zone offset to the UTC datetime value using DATEADD to obtain the corresponding datetime in the target time zone.
These examples demonstrate how to use DATEADD for basic time zone conversions in T-SQL. Keep in mind that these conversions may not account for daylight saving time changes or other nuances of time zone handling. For more robust time zone conversions, consider using a dedicated library or tool designed for this purpose.
Comments